Available with: All-In-One, EOD Historical Data — All World, EOD+Intraday — All World Extended and Free packages.
Consumption: Each request consumes 1 API call regardless of price history length.
FREE SUBSCRIPTIONS HAVE ACCESS TO 1 YEAR OF EOD DATA.
With End-of-Day data API, we have data for more than 150 000 tickers all around the world. We cover all US stocks, ETFs, and Mutual Funds (more than 51 000 in total) from the beginning, for example, the Ford Motors data is from Jun 1972 and so on. And non-US stock exchanges we cover mostly from Jan 3, 2000. We do provide daily, weekly and monthly data raw and adjusted to splits and dividends.
Quick jump:
Quick Start
To get historical stock price data use the following URL:
https://eodhd.com/api/eod/MCD.US?api_token=demo&fmt=json
curl --location "https://eodhd.com/api/eod/MCD.US?api_token=demo&fmt=json"
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://eodhd.com/api/eod/MCD.US?api_token=demo&fmt=json',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$data = curl_exec($curl);
curl_close($curl);
try {
$data = json_decode($data, true, 512, JSON_THROW_ON_ERROR);
var_dump($data);
} catch (Exception $e) {
echo 'Error. '.$e->getMessage();
}
import requests
url = f'https://eodhd.com/api/eod/MCD.US?api_token=demo&fmt=json'
data = requests.get(url).json()
print(data)
library(httr)
library(jsonlite)
url <- 'https://eodhd.com/api/eod/MCD.US?api_token=demo&fmt=json'
response <- GET(url)
if (http_type(response) == "application/json") {
content <- content(response, "text", encoding = "UTF-8")
cat(content)
} else {
cat("Error while receiving data\n")
}
- MCD.US consists of two parts: {SYMBOL_NAME}.{EXCHANGE_ID}, then you can use, for example, MCD.MX for Mexican Stock Exchange. or MCD.US for NYSE. Check the list of supported exchanges to get more information about the stock markets we do support.
- api_token – your own API KEY, which you will get after you subscribe to our services.
- fmt – the output format. Possible values are ‘csv’ for CSV output and ‘json’ for JSON output. Default value: ‘csv’.
- period – use ‘d’ for daily, ‘w’ for weekly, ‘m’ for monthly prices. By default, daily prices will be shown. Data aggregated weekly and monthly also shows weekly and monthly volume.
- order – use ‘a’ for ascending dates (from old to new), ‘d’ for descending dates (from new to old). By default, dates are shown in ascending order.
- from and to – the format is ‘YYYY-MM-DD’. If you need data from Jan 5, 2017, to Feb 10, 2017, you should use from=2017-01-05 and to=2017-02-10.
For testing purposes, you can try the following API Key (works only for MCD.US ticker): demo
https://eodhd.com/api/eod/MCD.US?period=d&api_token=demo&fmt=csv
curl --location "https://eodhd.com/api/eod/MCD.US?period=d&api_token=demo&fmt=csv"
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://eodhd.com/api/eod/MCD.US?period=d&api_token=demo&fmt=csv', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'GET', )); $data = curl_exec($curl); curl_close($curl); echo $data;
import requests url = f'https://eodhd.com/api/eod/MCD.US?period=d&api_token=demo&fmt=csv' data = requests.get(url).content print(data)
library(httr) library(jsonlite) url <- 'https://eodhd.com/api/eod/MCD.US?period=d&api_token=demo&fmt=csv' response <- GET(url) if (http_type(response) == "application/csv") { content <- content(response, "text", encoding = "UTF-8") cat(content) } else { cat("Error while receiving data\n") }
As a result, you will get the following data in CSV format:
Please note, that OHLC we provide in raw adjusted neither to splits nor to dividends, while adjusted closes are adjusted to both splits and dividends, and volume is adjusted to splits. If you need OHLC adjusted only to splits then it’s better to use our Technical API with the ‘function=splitadjusted’ parameter.
Daily Updates API
For daily updates on your end-of-day data, we recommend a special Bulk API for EOD, Splits and Dividends. With this API you will be able to download the data for a particular day for the entire exchange in seconds. Even to download the entire US exchange with more than 45,000 active tickers, you will need 1 API request and 5-10 seconds.
Stock Prices Data API with Dates Support
We support two formats for historical data dates.
EOD Historical Data
Here you can use ‘from’ and ‘to’ parameters with the format ‘YYYY-MM-DD’. For example, if you need to get data only from Jan 5, 2017, to Feb 10, 2017, you need to use from=2017-01-05 and to=2017-02-10. Then the final URL will be:
https://eodhd.com/api/eod/MCD.US?from=2020-01-05&to=2020-02-10&period=d&api_token=demo&fmt=json
curl --location "https://eodhd.com/api/eod/MCD.US?from=2020-01-05&to=2020-02-10&period=d&api_token=demo&fmt=json"
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://eodhd.com/api/eod/MCD.US?from=2020-01-05&to=2020-02-10&period=d&api_token=demo&fmt=json',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$data = curl_exec($curl);
curl_close($curl);
try {
$data = json_decode($data, true, 512, JSON_THROW_ON_ERROR);
var_dump($data);
} catch (Exception $e) {
echo 'Error. '.$e->getMessage();
}
import requests
url = f'https://eodhd.com/api/eod/MCD.US?from=2020-01-05&to=2020-02-10&period=d&api_token=demo&fmt=json'
data = requests.get(url).json()
print(data)
library(httr)
library(jsonlite)
url <- 'https://eodhd.com/api/eod/MCD.US?from=2020-01-05&to=2020-02-10&period=d&api_token=demo&fmt=json'
response <- GET(url)
if (http_type(response) == "application/json") {
content <- content(response, "text", encoding = "UTF-8")
cat(content)
} else {
cat("Error while receiving data\n")
}
Filter fields. WEBSERVICE and YAHOO Support
We also support ‘=WEBSERVICE’ Excel function, to get only the last value, just add ‘filter=last_close’ or ‘filter=last_volume’ with ‘fmt=json’ and you will get only one number which perfectly works with WEBSERVICE function. For example, with this URL:
https://eodhd.com/api/eod/MCD.US?filter=last_close&api_token=demo&fmt=json
curl --location "https://eodhd.com/api/eod/MCD.US?filter=last_close&api_token=demo&fmt=json"
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://eodhd.com/api/eod/MCD.US?filter=last_close&api_token=demo&fmt=json',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$data = curl_exec($curl);
curl_close($curl);
try {
$data = json_decode($data, true, 512, JSON_THROW_ON_ERROR);
var_dump($data);
} catch (Exception $e) {
echo 'Error. '.$e->getMessage();
}
import requests
url = f'https://eodhd.com/api/eod/MCD.US?filter=last_close&api_token=demo&fmt=json'
data = requests.get(url).json()
print(data)
library(httr)
library(jsonlite)
url <- 'https://eodhd.com/api/eod/MCD.US?filter=last_close&api_token=demo&fmt=json'
response <- GET(url)
if (http_type(response) == "application/json") {
content <- content(response, "text", encoding = "UTF-8")
cat(content)
} else {
cat("Error while receiving data\n")
}
You will get one value – the last price for MCD.
Yahoo Finance API Support
To support clients who used Yahoo Finance service non-official API which doesn’t work now (URL: ichart.finance.yahoo.com), we also support yahoo-style for dates. Here there will be 6 parameters.
- First of all, for yahoo-style you need to use another endpoint:
- https://eodhd.com/api/table.csv.
- For symbol you should use s, then for MCD it will be: s=MCD.US.
- For ‘from date’ you should use a, b, c for month, day, year. Then 2017-01-05 will be: a=00, b=05, c=2017.
- For ‘to date’ you should use d, e, f for month, day, year. Then 2017-02-10 will be: d=01, e=10, f=2017.
- Please note, that in Yahoo! Finance API first month is 0 and last month (December) is 11. Then you need to substract 1 from months.
- For ‘period’ you should use g. Possible values are d for daily, w for weekly and m for montly.
BE CAREFUL, date used here in American notation: MONTH, DAY, YEAR.
And the final URL will be:
https://eodhd.com/api/table.csv?s=MCD.US&a=05&b=01&c=2017&d=10&e=02&f=2017&g=d&api_token=demo&fmt=json
curl --location "https://eodhd.com/api/table.csv?s=MCD.US&a=05&b=01&c=2017&d=10&e=02&f=2017&g=d&api_token=demo&fmt=json"
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://eodhd.com/api/table.csv?s=MCD.US&a=05&b=01&c=2017&d=10&e=02&f=2017&g=d&api_token=demo&fmt=json',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$data = curl_exec($curl);
curl_close($curl);
try {
$data = json_decode($data, true, 512, JSON_THROW_ON_ERROR);
var_dump($data);
} catch (Exception $e) {
echo 'Error. '.$e->getMessage();
}
import requests
url = f'https://eodhd.com/api/table.csv?s=MCD.US&a=05&b=01&c=2017&d=10&e=02&f=2017&g=d&api_token=demo&fmt=json'
data = requests.get(url).json()
print(data)
library(httr)
library(jsonlite)
url <- 'https://eodhd.com/api/table.csv?s=MCD.US&a=05&b=01&c=2017&d=10&e=02&f=2017&g=d&api_token=demo&fmt=json'
response <- GET(url)
if (http_type(response) == "application/json") {
content <- content(response, "text", encoding = "UTF-8")
cat(content)
} else {
cat("Error while receiving data\n")
}
We hope it will be very useful for you.
JSON Output Support
We support JSON output as well if you need it for your PHP, Python, Java, or Perl applications. All you need is to add a special parameter: “fmt=json” to your query, then the final query will be:
https://eodhd.com/api/eod/MCD.US?from=2017-01-05&to=2017-02-10&period=d&api_token=demo&fmt=json
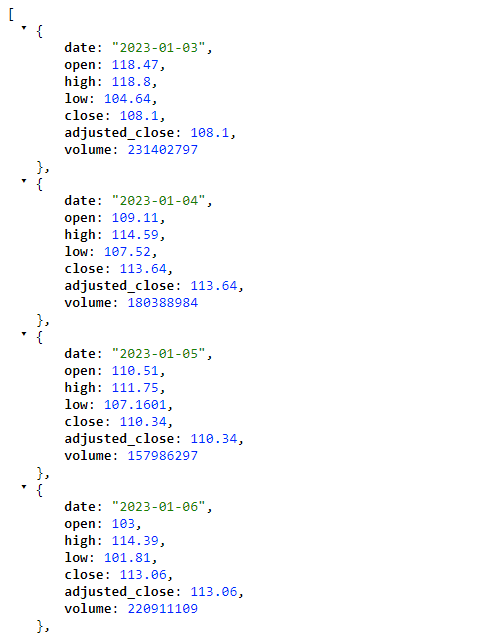
JSON output doesn’t work for Yahoo-like style.
We have API limits 100 000 requests per day. Each symbol request costs 1 API call.
End Of Day Historical Prices Update Time
We update each stock exchange 2-3 hours after the market closes. But except for major US exchanges (NYSE, NASDAQ), these exchanges are updated next 15 minutes after the market closes. However US mutual funds, PINK, OTCBB, and some indices do update only the next morning, the update starts at 3-4 am EST and usually ends at 5-6 am EST. For these types of symbols, we always get ‘updated price’ up to 3-4 am.