Available with: All-In-One, EOD+Intraday — All World Extended packages.
Consumption: Each request consumes 5 API calls.
We support intraday historical data for major exchanges all around the world.
Quick Start
IMPORTANT: data for all exchanges is provided in the UTC timezone, with Unix timestamps.
- For the US 1-minute interval data, only NYSE and NASDAQ tickers are supported, including pre-market (premarket) and after-hours (afterhours) trading data from 2004, more than 17 years of data. 5-minute and 1-hour intervals for US tickers are available from October 2020.
- For FOREX, Cryptocurrencies (CC), and MOEX (MCX) tickers, we have 1-minute intervals of trading data from 2009, more than 12 years of data. 5-minute and 1-hour intervals are available from October 2020.
- For most other tickers from most other exchanges, we have 5-minute and 1-hour intervals from October 2020. Some of these tickers may also have 1-minute interval data from October 2020, but the availability of this data varies between tickers and exchanges.
The data is historical, updated 2-3 hours after market closing.
To get intraday historical stock price data use the following URL:
URL
cURL
PHP
Python
R
https://eodhd.com/api/intraday/AAPL.US?api_token=demo&fmt=json
curl --location "https://eodhd.com/api/intraday/AAPL.US?api_token=demo&fmt=json"
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://eodhd.com/api/intraday/AAPL.US?api_token=demo&fmt=json',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$data = curl_exec($curl);
curl_close($curl);
try {
$data = json_decode($data, true, 512, JSON_THROW_ON_ERROR);
var_dump($data);
} catch (Exception $e) {
echo 'Error. '.$e->getMessage();
}
import requests
url = f'https://eodhd.com/api/intraday/AAPL.US?api_token=demo&fmt=json'
data = requests.get(url).json()
print(data)
library(httr)
library(jsonlite)
url <- 'https://eodhd.com/api/intraday/AAPL.US?api_token=demo&fmt=json'
response <- GET(url)
if (http_type(response) == "application/json") {
content <- content(response, "text", encoding = "UTF-8")
cat(content)
} else {
cat("Error while receiving data\n")
}
- AAPL.US consists of two parts: {SYMBOL_NAME}.{EXCHANGE_ID}, then you can use, for example, AAPL.MX for Mexican Stock Exchange. or AAPL.US for NASDAQ. Check the list of supported exchanges to get more information about the stock markets we support.
- api_token – your own API KEY, which you will get after subscribing to our services.
- interval – the possible intervals: ‘5m’ for 5-minutes, ‘1h’ for 1 hour, and ‘1m’ for 1-minute intervals.
- fmt – use this parameter to get the data in a different format. Possible values are ‘json’ for JSON and ‘csv’ for CSV. By default, the data is provided in CSV format.
- from and to – use these parameters to filter data by datetime. Parameters should be passed in UNIX time with UTC timezone, for example, these values are correct: “from=1627896900&to=1630575300” and correspond to ‘ 2021-08-02 09:35:00 ‘ and ‘ 2021-09-02 09:35:00 ‘. The maximum periods between ‘from’ and ‘to’ are 120 days for 1-minute intervals, 600 days for 5-minute intervals and 7200 days for 1-hour intervals (please note, especially with the 1-hour interval, this is the maximum theoretically possible length). Without ‘from’ and ‘to’ specified, the length of the data obtained is the last 120 days.
For testing purposes you can try the following API Key (works only for AAPL.US ticker): demo
URL
cURL
PHP
Python
R
https://eodhd.com/api/intraday/AAPL.US?interval=5m&api_token=demo&fmt=csv
curl --location "https://eodhd.com/api/intraday/AAPL.US?interval=5m&api_token=demo&fmt=csv"
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://eodhd.com/api/intraday/AAPL.US?interval=5m&api_token=demo&fmt=csv', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'GET', )); $data = curl_exec($curl); curl_close($curl); echo $data;
import requests url = f'https://eodhd.com/api/intraday/AAPL.US?interval=5m&api_token=demo&fmt=csv' data = requests.get(url).content print(data)
library(httr) library(jsonlite) url <- 'https://eodhd.com/api/intraday/AAPL.US?interval=5m&api_token=demo&fmt=csv' response <- GET(url) if (http_type(response) == "application/csv") { content <- content(response, "text", encoding = "UTF-8") cat(content) } else { cat("Error while receiving data\n") }
As a result, you will get the following data in CSV format:
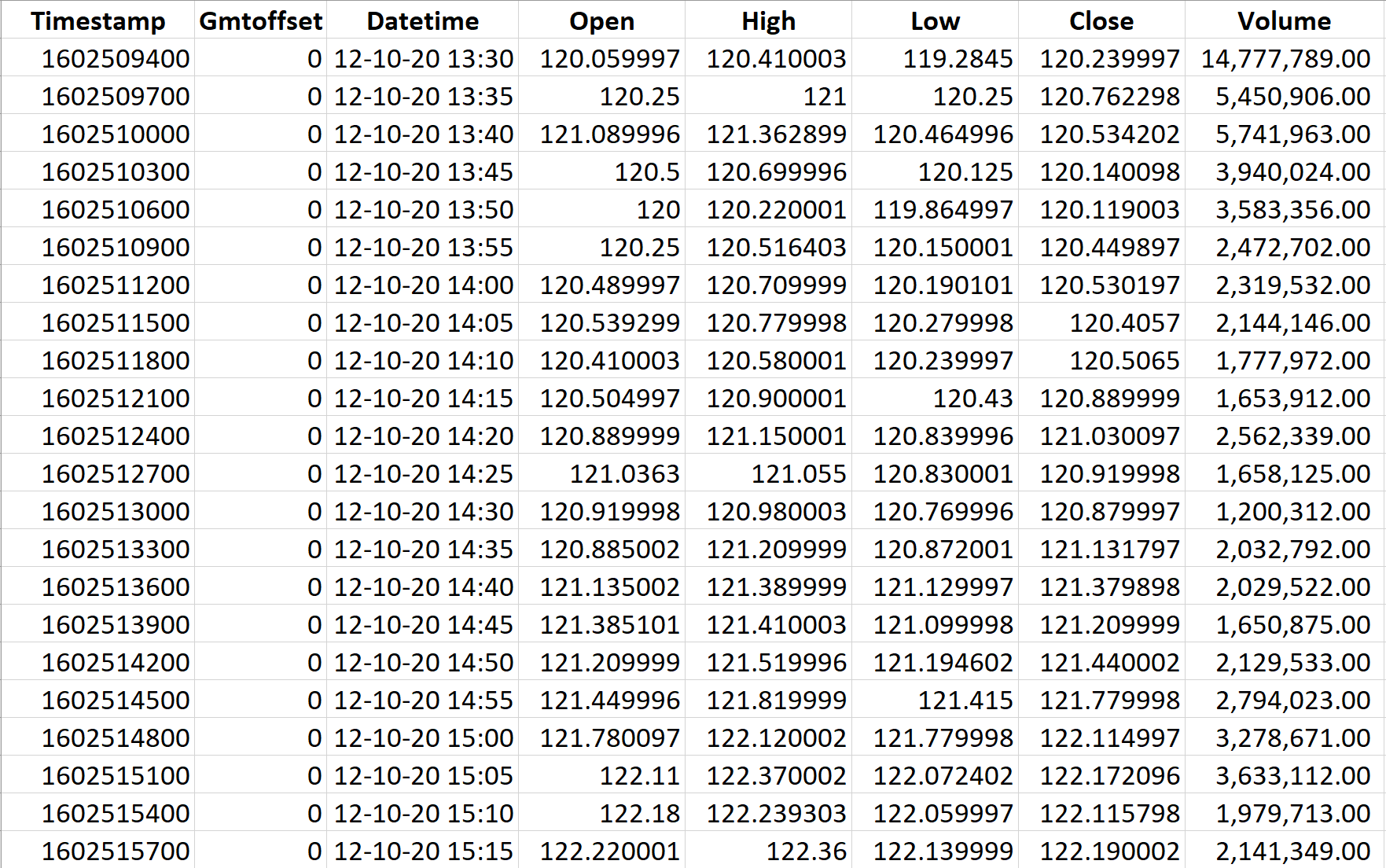
We have API limits 100 000 requests per day per subscription. Each symbol request costs 5 API calls.