Available with: All-In-One, EOD Historical Data — All World, EOD+Intraday — All World Extended, Fundamentals Data Feed and Free packages.
Consumption: Each request consumes 1 API call.
Get List of Exchanges
We support more than 60 exchanges all around the world. All US exchanges are combined into one virtual exchange ‘US,’ which includes NYSE, NASDAQ, NYSE ARCA, and OTC/PINK tickers.
To get the full list of supported exchanges with names, codes, operating MICs, country, and currency, you can use the ‘exchanges-list’ endpoint:
https://eodhd.com/api/exchanges-list/?api_token={YOUR_API_TOKEN}&fmt=json
curl --location "https://eodhd.com/api/exchanges-list/?api_token={YOUR_API_TOKEN}&fmt=json"
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://eodhd.com/api/exchanges-list/?api_token={YOUR_API_TOKEN}&fmt=json',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$data = curl_exec($curl);
curl_close($curl);
try {
$data = json_decode($data, true, 512, JSON_THROW_ON_ERROR);
var_dump($data);
} catch (Exception $e) {
echo 'Error. '.$e->getMessage();
}
import requests
url = f'https://eodhd.com/api/exchanges-list/?api_token={YOUR_API_TOKEN}&fmt=json'
data = requests.get(url).json()
print(data)
library(httr)
library(jsonlite)
url <- 'https://eodhd.com/api/exchanges-list/?api_token={YOUR_API_TOKEN}&fmt=json'
response <- GET(url)
if (http_type(response) == "application/json") {
content <- content(response, "text", encoding = "UTF-8")
cat(content)
} else {
cat("Error while receiving data\n")
}
There are no additional parameters, an example output can be found below:
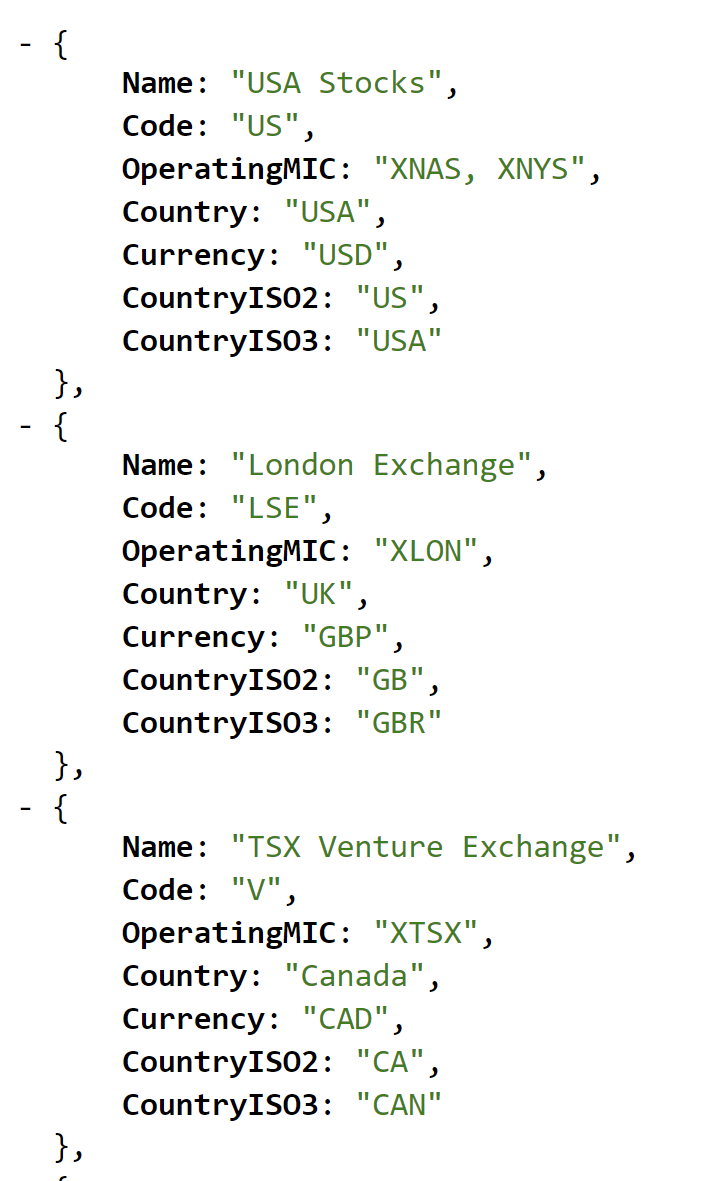
Get List of Tickers (Exchange Symbols)
To get the list of tickers for an exchange, you need to be authenticated and have a proper API key with access to this exchange. The URL will look like this:
https://eodhd.com/api/exchange-symbol-list/{EXCHANGE_CODE}?api_token={YOUR_API_TOKEN}&fmt=json
curl --location "https://eodhd.com/api/exchange-symbol-list/{EXCHANGE_CODE}?api_token={YOUR_API_TOKEN}&fmt=json"
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://eodhd.com/api/exchange-symbol-list/{EXCHANGE_CODE}?api_token={YOUR_API_TOKEN}&fmt=json', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'GET', )); $data = curl_exec($curl); curl_close($curl); try { $data = json_decode($data, true, 512, JSON_THROW_ON_ERROR); var_dump($data); } catch (Exception $e) { echo 'Error. '.$e->getMessage(); }
import requests url = f'https://eodhd.com/api/exchange-symbol-list/{EXCHANGE_CODE}?api_token={YOUR_API_TOKEN}&fmt=json' data = requests.get(url).json() print(data)
library(httr) library(jsonlite) url <- 'https://eodhd.com/api/exchange-symbol-list/{EXCHANGE_CODE}?api_token={YOUR_API_TOKEN}&fmt=json' response <- GET(url) if (http_type(response) == "application/json") { content <- content(response, "text", encoding = "UTF-8") cat(content) } else { cat("Error while receiving data\n") }
By default, this API provides only tickers that were active at least a month ago, to get the list of inactive (delisted) tickers please use the parameter “delisted=1”:
https://eodhd.com/api/exchange-symbol-list/{EXCHANGE_CODE}?delisted=1&api_token={YOUR_API_TOKEN}&fmt=json
curl --location "https://eodhd.com/api/exchange-symbol-list/{EXCHANGE_CODE}?delisted=1&api_token={YOUR_API_TOKEN}&fmt=json"
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://eodhd.com/api/exchange-symbol-list/{EXCHANGE_CODE}?delisted=1&api_token={YOUR_API_TOKEN}&fmt=json', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'GET', )); $data = curl_exec($curl); curl_close($curl); try { $data = json_decode($data, true, 512, JSON_THROW_ON_ERROR); var_dump($data); } catch (Exception $e) { echo 'Error. '.$e->getMessage(); }
import requests url = f'https://eodhd.com/api/exchange-symbol-list/{EXCHANGE_CODE}?delisted=1&api_token={YOUR_API_TOKEN}&fmt=json' data = requests.get(url).json() print(data)
library(httr) library(jsonlite) url <- 'https://eodhd.com/api/exchange-symbol-list/{EXCHANGE_CODE}?delisted=1&api_token={YOUR_API_TOKEN}&fmt=json' response <- GET(url) if (http_type(response) == "application/json") { content <- content(response, "text", encoding = "UTF-8") cat(content) } else { cat("Error while receiving data\n") }
For US exchanges, you can get all US tickers combined into one list by using the ‘US’ exchange code. Tickers for individual exchanges are obtained with the following exchange codes:
'NYSE', 'NASDAQ', 'BATS', 'OTCQB', 'PINK', 'OTCQX', 'OTCMKTS', 'NMFQS', 'NYSE MKT','OTCBB', 'OTCGREY', 'BATS', 'OTC'
It is possible to use an additional parameter to filter the tickers by type:
- type – filters the output by ticker type. Possible types: common_stock, preferred_stock, stock (both common and preferred combined), etf, fund. E. g.: adding &type=etf to your request will return all ETFs of the given exchange.
We support both CSV and JSON output for this API endpoint. As output, you will get the following information: Symbol (or Code), Company Name, Country, Exchange, and Currency. And here is a sample output for list symbols of exchange:
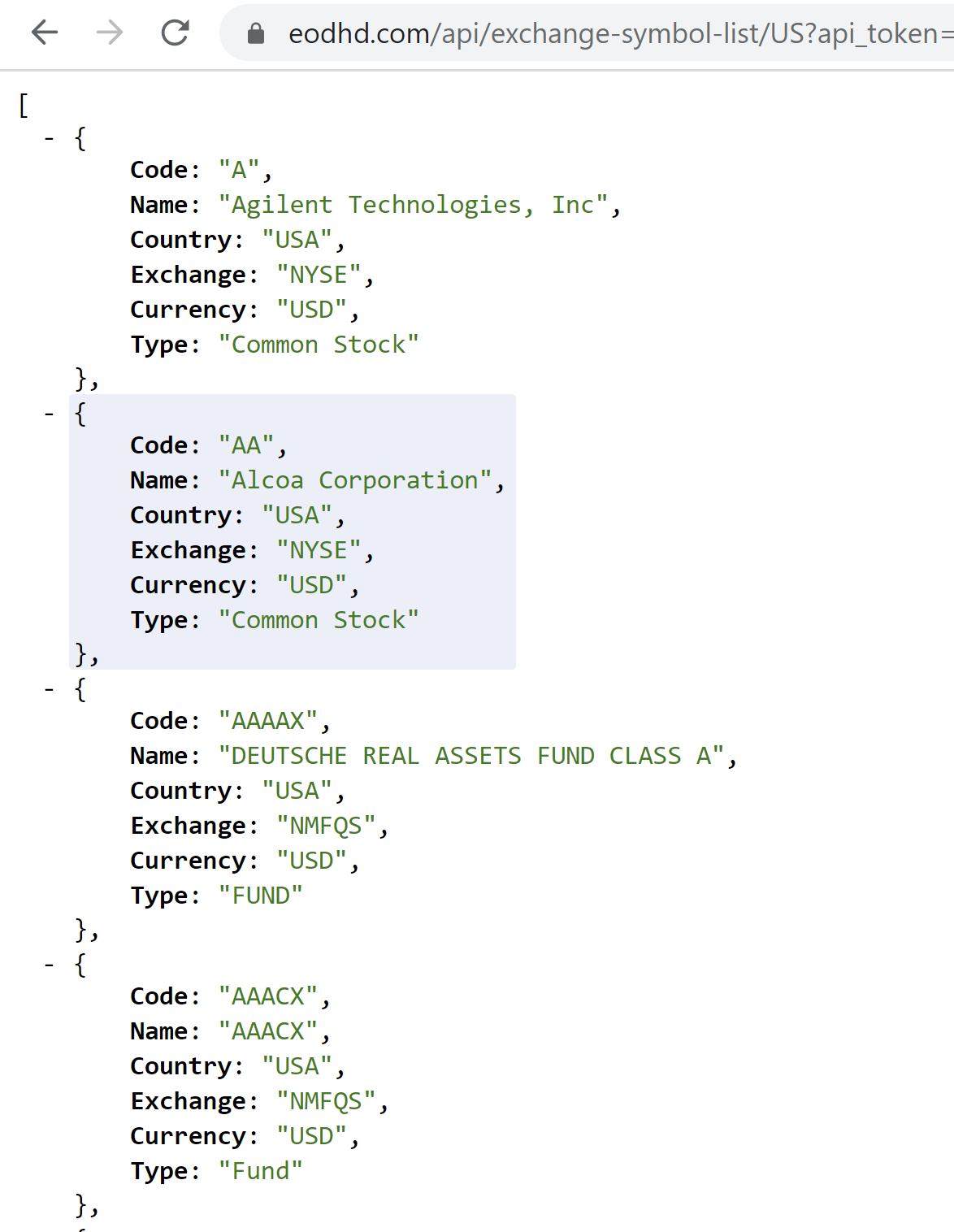
You can also get the full list of supported tickers with our Sitemap.
And don’t forget to check our Exchange Trading Hours and Holidays API!