Python is one of the most popular programming languages, especially when dealing with vast amounts of financial data. Whether you’re developing a standalone app for finance or applying analytical methods to financial data, Python offers numerous solutions. While tools like Google Sheets and Excel add-ons cater to those less familiar with coding, we’ve made significant efforts to enhance the lives of developers by providing EODHD’s official library for API for Python to pull financial statement data and many more. In this article, we will cover installation, all functions, and provide examples, catering to developers in the finance industry who seek the best solutions for various tasks, including scraping financial data from several sources.
Quick jump:
Official EODHD Python Financial Library installation
The library grants access to all the key features to work with stock market API in Python. You can find the library with it’s updates on our Github page.
1. Installing EODHD library. Start with the following command:
python3 -m pip install eodhd -U
See the screenshot:

Wait until library will be installed – it will be shown when [*] will change to [1].
2. Importing Required Libraries. Next, let’s import EODHD library for accessing EODHD API’s functions:
from eodhd import APIClient
import pandas as pd
Python Financial Library is installed now.
3. Accessing the EODHD API – to access the EODHD API, we need to create an instance of the “APIClient” class and pass it our API key. In this example, we will use the demo API key provided by EODHD, but in a real application, you should use your registered API key.
api = APIClient("<Your_API_Key>")
or:
import os
api_key = os.environ.get("<Your_API_Key>")
api = APIClient(api_key)
or via config file:
# config.py
API_KEY = "<Your_API_Key>"
# main.py
import config as cfg
api = APIClient(cfg.API_KEY)
There is also an unofficial, community-built Python library for EODHD’s API by Lautaro Parada. The library and its description can be found on the GitHub page.
Next, we are going to activate EODHD’s API key to get an access to stock data API in Python.
EODHD API access activation: free and payed options
1. You can start with “DEMO” API key to test the data for a few tickers only: AAPL.US, TSLA.US , VTI.US, AMZN.US, BTC-USD and EUR-USD. For these tickers, all of our types of data (APIs), including Real-Time Data, are available without limitations.
2. Register for the free plan to receive your API key (limited to 20 API calls per day) with access to End-Of-Day Historical Stock Market Data API for any ticker, but within the past year only. Plus a List of tickers per Exchange is available.
3. We recommend to explore our plans, starting from $19.99, to access the necessary type of data without limitations.
The library is now ready for use. Next, we recommend exploring our Academy section and specifically the article “Download EOD, Intraday, and Real-time Prices for Any Cryptocurrency with Python Simply” by Michael Whittle to fully understand what our API offers.
Example: End of the day historical stock market data
Lets retrieve End of the day historical stock market data:
resp = api.get_eod_historical_stock_market_data(symbol = 'AAPL.MX', period='d', from_date = '2023-01-01', to_date = '2023-01-15', order='a')
print(resp)
The function will return the following JSON respond:
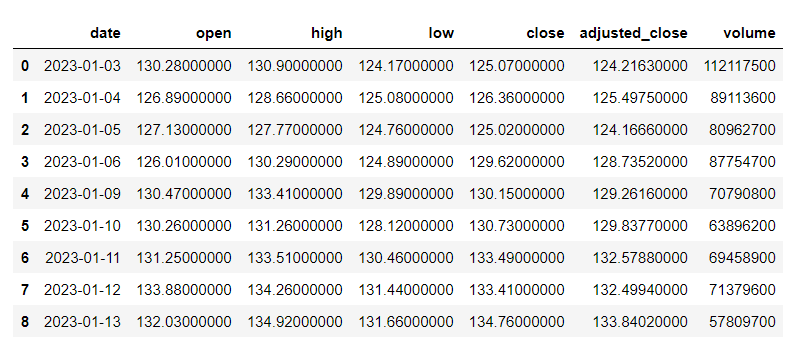
All the parameters for this function are listed and described here.
List of the available functions
Function & description | Code examples |
---|---|
Live (Delayed) Stock Prices and Macroeconomic Data (doc) |
|
Bonds Fundamentals (doc) |
|
Intraday Historical Data (doc) |
|
Historical Dividends (doc) |
|
Historical Splits (doc) |
|
Bulk API for EOD, Splits and Dividends (doc) |
|
Calendar. Upcoming Earnings, Trends, IPOs and Splits (doc) |
|
Economic Events (doc) |
|
Stock Market and Financial News (doc) |
|
End of the Day Historical Stock Market Data (doc) |
|
List of supported Exchanges (doc) |
|
Insider Transactions (doc) |
|
Macro Indicators (doc) |
|
Exchanges API. Trading Hours, Stock Market Holidays, Symbols Change History (doc) |
|
Stock Market Screener (doc) |
|
Technical Indicator (doc) |
|
Historical Market Capitalization (doc) |
|
Fundamental Data: Stocks, ETFs, Mutual Funds, Indices and Cryptocurrencies (doc) |
|