Available with: All-In-One, EOD Historical Data — All World, EOD+Intraday — All World Extended and Free packages.
Consumption: Each request consumes 1 API call per ticker.
EODHD APIs offer one of the best ways for trading enthusiasts, developers, and data analysts to incorporate live financial data into their decision-making projects, with a delay of 15-20 min covering almost all stocks on the market and 1 min delay for 1100+ Forex pairs.
To mention, our stock market data APIs offer Real-time and Live (delayed data). Real-time data API, with a 50ms delay, is based on the WebSocket protocol, providing access to the US stock market, FOREX, and alternative currencies, and is included in two payed pricing plans. Meanwhile, the Live (15-20 min delay for stocks and 1 min delay for Forex pairs) API, described in this article, is included in both free and paid plans, offering access to US and global delayed stock quotes API.
1. You can start with “DEMO” API key to test the data for a few tickers only: AAPL.US, TSLA.US , VTI.US, AMZN.US, BTC-USD and EUR-USD. For these tickers, all of our types of data (APIs), including Real-Time Data, are available without limitations.
2. Register for the free plan to receive your API key (limited to 20 API calls per day) with access to End-Of-Day Historical Stock Market Data API for any ticker, but within the past year only. Plus a List of tickers per Exchange is available.
3. We recommend to explore our plans, starting from $19.99, to access the necessary type of data without limitations.
Quick jump:
Live Stock Market API features
- A support for almost all symbols and exchanges worldwide
- Prices are provided with a 15-20 minute delay
- Data with a 1-minute interval, meaning you receive prices at 1-minute frequency
- Multiple tickers can be requested with a single request
- Excel Webservice support
- JSON and CSV formats
Live request example
Here is an example of live (delayed) stock prices API for AAPL (Apple Inc). Please note that the API token ‘demo’ only works for AAPL.US, TSLA.US , VTI.US, AMZN.US, BTC-USD tickers:
https://eodhd.com/api/real-time/AAPL.US?api_token=demo&fmt=json
curl --location "https://eodhd.com/api/real-time/AAPL.US?api_token=demo&fmt=json"
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://eodhd.com/api/real-time/AAPL.US?api_token=demo&fmt=json',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$data = curl_exec($curl);
curl_close($curl);
try {
$data = json_decode($data, true, 512, JSON_THROW_ON_ERROR);
var_dump($data);
} catch (Exception $e) {
echo 'Error. '.$e->getMessage();
}
import requests
url = f'https://eodhd.com/api/real-time/AAPL.US?api_token=demo&fmt=json'
data = requests.get(url).json()
print(data)
library(httr)
library(jsonlite)
url <- 'https://eodhd.com/api/real-time/AAPL.US?api_token=demo&fmt=json'
response <- GET(url)
if (http_type(response) == "application/json") {
content <- content(response, "text", encoding = "UTF-8")
cat(content)
} else {
cat("Error while receiving data\n")
}
JSON response is:
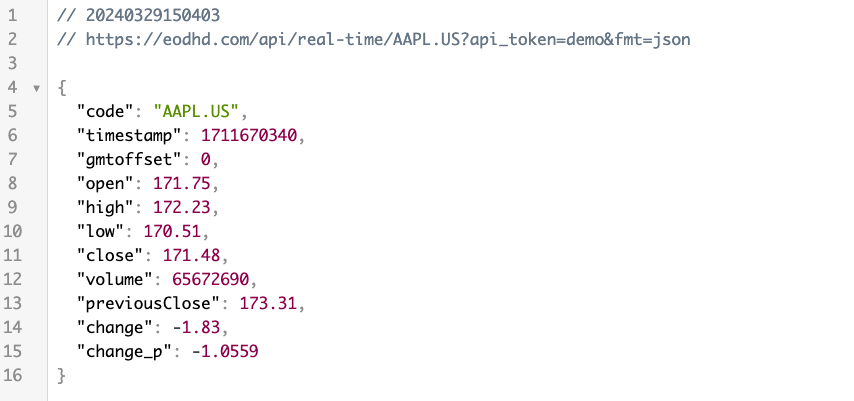
Timestamp field explanation
We use Unix time for our timestamp field. Unix time represents a point in time by counting the number of seconds that have passed since 00:00:00 Coordinated Universal Time (UTC) on Thursday, January 1, 1970. You can easily check and convert all our timestamps using this tool.
For example, in the response above, the timestamp 1711670340 corresponds to Thursday, March 28, 2024, 23:59:00 GMT.
Multiple Tickers with One Request
Add the ‘s=’ parameter to your URL, and you will be able to retrieve data for multiple tickers in a single request. All tickers should be separated by a comma. For example, you can request data for AAPL.US, VTI, and EUR.FOREX with the following URL:
https://eodhd.com/api/real-time/AAPL.US?s=VTI,EUR.FOREX&api_token=demo&fmt=json
curl --location "https://eodhd.com/api/real-time/AAPL.US?s=VTI,EUR.FOREX&api_token=demo&fmt=json"
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://eodhd.com/api/real-time/AAPL.US?s=VTI,EUR.FOREX&api_token=demo&fmt=json',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$data = curl_exec($curl);
curl_close($curl);
try {
$data = json_decode($data, true, 512, JSON_THROW_ON_ERROR);
var_dump($data);
} catch (Exception $e) {
echo 'Error. '.$e->getMessage();
}
import requests
url = f'https://eodhd.com/api/real-time/AAPL.US?s=VTI,EUR.FOREX&api_token=demo&fmt=json'
data = requests.get(url).json()
print(data)
library(httr)
library(jsonlite)
url <- 'https://eodhd.com/api/real-time/AAPL.US?s=VTI,EUR.FOREX&api_token=demo&fmt=json'
response <- GET(url)
if (http_type(response) == "application/json") {
content <- content(response, "text", encoding = "UTF-8")
cat(content)
} else {
cat("Error while receiving data\n")
}
Please note that ‘AAPL.US’ is used here at the beginning, and additional tickers are added with the ‘s=’ parameter.
We recommend limiting the number of tickers per request to 15-20 for optimal performance.
JSON and CSV formats
We support live (delayed) stock price data in both JSON and CSV formats. If you prefer CSV output, simply change ‘fmt=json’ to ‘fmt=csv’ or remove this parameter altogether.
Daily limits
Our API has a limit of 100,000 requests per day, with each symbol request counting as 1 API call. For instance, a request for multiple tickers with 10 symbols will consume 10 API calls.
Live data via Excel and Google Sheets
Access live data through our free Excel and Google Sheets add-ons. Learn more about them and download them here. With these add-ons, financial data for stocks, ETFs, mutual funds, and FOREX markets is now available on any device without coding. Additionally, we provide Excel support for Webservice.
Conclusion
EODHD provides one of the best solutions for integrating live finance data into decision-making projects, offering stock market data with real-time streaming and historical data API capabilities across global markets. Explore our pricing plans to explore all avalible features for your financial projects. We recommend checking out our Live (Delayed) Data API, which complements our offerings with free real-time and historical stock market data, empowering users with insights for their trading strategies.